Project Structure
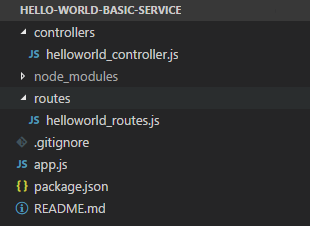
There are three major files
App.js
This is a very basic file, you can change the Port number if you want here.
Routes
The routes/helloworld_routes.js file has the mapping between the path and the request handler.
Controller
The controllers/helloworld_controller.js file has the methods for handling the request.
app.js
'use strict';
var express = require('express');
var bodyParser = require('body-parser');
var http = require('http');
var httpServer;
var app = express();
app.use(bodyParser.json());
app.use(bodyParser.urlencoded({extended: true}));
var routes = [
"helloworld_routes"
]
function setupRoutes(){
for(var route of routes){
app.use('/' , require("./routes/"+ route));
}
}
function startServer() {
try{
var portnumber =8888;
setupRoutes();
httpServer = http.createServer(app).listen(portnumber, '0.0.0.0');
console.log("Server started at port "+portnumber);
console.log("URL http://localhost:"+portnumber);
}
catch(err){
console.log("app.js startServer :: err :: ", err);
}
}
startServer();
helloworld_routes.js
'use strict';
var express = require('express');
var helloWorldRouter = require('../controllers/helloworld_controller.js');
var router = module.exports = express.Router();
router.post("/hello", helloWorldRouter.helloworld);
router.get("/hello", helloWorldRouter.helloworld);
helloworld_controller.js
'use strict';
module.exports = {
helloworld : helloworld
}
function helloworld(request, response) {
response.status(200).send({ data : "Hello World"});
}
